Author:
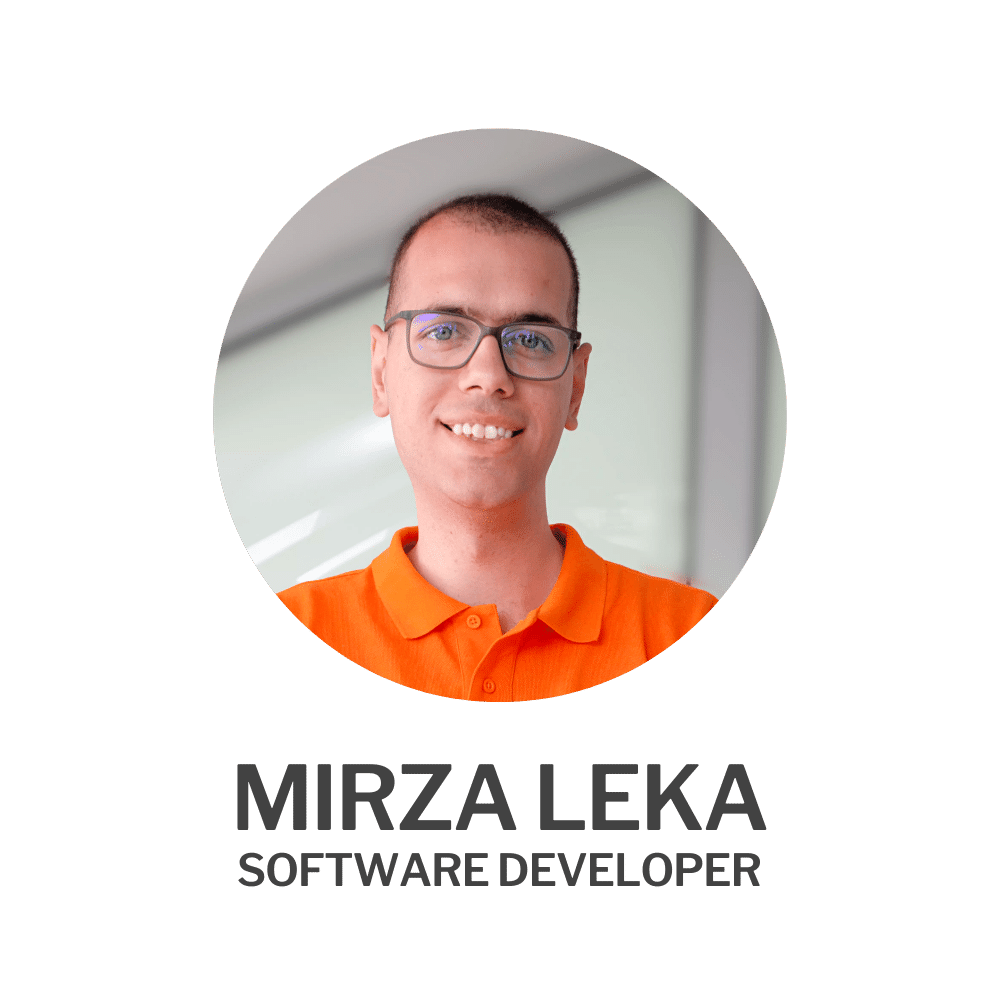
Tips and Tricks to improve the way you write JavaScript.
In simple terms, it's any value that evaluates to false, nullable, or empty.
What this means is that if you have a variable:
const name = '';
And a condition where you want to check the variable:
if (CHECK IF NAME IS EMPTY) { throw new Error('Missing name'); }
You do not need to compare it to an empty string or null or undefined:
// Unnecessary if (name === '' || name === undefined || name === null) { ... }
Or convert it to a boolean to validate if it's Falsy (as you'd in other strongly typed languages).
Instead, consider it as a Falsy value (like a boolean) and just put
! prefix and JavaScript will evaluate it for you:
// Simpler if (!name) { throw new Error('Missing name'); }
What is Truthy? Well, the opposite of Falsy:
// name = ''; !name === !false // True // name = undefined; !name === !false // True // name = null; !name === !false // True // name = []; !name.length === !false // True // name = 0 !name === !false // True
Likewise, if the outcome is the opposite, it will evaluate to a Truthy value.
// sport = 'Football'; !sport === !true // True // sport = ' '; !sport === !true // True // sport = ['Football']; !sport.length === !true // True // sport = 1 !sport === !true // True
A common way to toggle between truthy and falsy values is to use the logical operators:
When setting up a condition between values, the && operator will capture a value that evaluates to False.
If either value in a condition is Falsy, the output will be false:
true && false // False false && true // False false && false // False
Only if both values are Truthy, the && operator will evaluate to true:
true && true // True
Keep in mind that && will evaluate all values in a condition until it finds the first Falsy value. Otherwise, it will take the last value.
true && 'Hello' && 100; // 100 (Truthy value)
If Either condition fails, the statement will be evaluated as False.
const email = 'hello'; if (email.includes('@') && email.includes('.com') { console.log('Email is valid!'); } else { console.log('Email is invalid!'); }
In Web frameworks like React, you can display HTML elements (or components) only when a certain condition is satisfied.
<div> { conditionIsMet && <h1>Hello World</h1> } </div>
A common mistake that developers make is to attempt to read a value from a property whose parent is null/undefined.
const array = []; console.log(array.length); ✔️ const newArray = null; console.log(newArray.length); ❌ // Exception
To solve this, you first make sure that the root property is not null/undefined and then try to read the contents of a given property.
if (newArray !== null && newArray.length === 0) { console.log('newArray is empty!'); }
The logical OR (||) operator works the opposite of the && operator. It looks for any value in a condition that results in Truthy and takes that value.
true || true // True true || false // True false || true // True
Only if both values are Falsy, the outcome will be false:
false || false // False
|| will evaluate all values in a condition until it finds the first Truthy value. Otherwise, it will take the last value.
false || undefined || null // Null
The || operator can be used to filter items that meet one condition or another.
const clothes = [ { type: 'Shirt', color: 'Black', size: 'XL', price: 0 }, { type: 'Shirt', color: 'White', size: 'XL', price: 100 }, { type: 'Skirt', color: 'Red', size: 'L', price: 50 } ]; const favClothes = clothes .filter(item => item.color === 'Black' || item.color === 'White'); console.log('favClothes :>> ', favClothes); /* [ { type: 'Shirt', color: 'Black', size: 'XL' }, { type: 'Shirt', color: 'White', size: 'XL' } ] */
If one expression turns out Falsy, you can use the || operator to set up a default value:
function findHero(hero) { const defaultHero = 'Goku'; const myHero = hero || defaultHero; return heroes.find(h => h.name === myHero); }
When you have multiple && & || operators in a single statement, the rule of precedence applies. The first two conditions will be evaluated and then the outcome of that against the third value;
console.log(true && null || false ); // False // #1 true && null (where null is Falsy) === Null // #2 null || false === False
console.log(false || 100 && undefined ); // Undefined // #1 false || 100 (where 100 is Truthy) === 100 // #2 100 && undefined (truthy && falsy) === Undefined
console.log(null || 0 && '' || true); // True console.log(null || 0 && '' && false); // 0
The ES2020 introduced a new operator, the Nullish Coalescing, also known as the Elvis operator.
const possiblyNull = null; const result = possiblyNull ?? 'Default Value'; console.log(result); // 'Default Value'
const possiblyUndefined = undefined; const result = possiblyUndefined ?? 'Default Value'; console.log(result); // 'Default Value'
It works similarly to the || operator. However, while || applies to all falsy values, the Nullish Coalescing operator is limited to only null and undefined values.
If used against other types, it will just return the first value:
false ?? true // False true ?? false // True
Another way to avoid nullable properties is to use the Optional Chaining operator.
Instead of repeating yourself:
if (newArray !== null && newArray.length === 0) { console.log('newArray is empty!'); }
You can apply the Optional Chaining:
if (newArray?.length === 0) { console.log('newArray is empty!'); }
What this will do is:
And it's much cleaner.
Also works for as many properties as you have:
const name = currentUser?.username?.name?.firstName;
You can combine the Optional Chaining with other operators as well:
The 'Default Username' will print if:
Alternatively, assuming the user property is already defined (has value), you can assign a default value to user.username using the Object Destructuring:
Where username will equal to 'Default Username' only if it's undefined or null.
A common inline way to toggle between truthy and falsy values is to use the Ternary Operator. It works like:
const isGreaterThan = 10 > 1 ? true : false; console.log(isGreaterThan); // True // const isLesserThan = 10 < 1 ? true : false; console.log(isLesserThan); // False
The latest addition to JavaScript is a set of Logical Assignment operators. Think of everything you've learned so far combined with an ability to assign values on the fly.
So instead of doing:
function setHero(myHero) { myHero = myHero ?? 'Goku'; return myHero; } const result = setHero(); console.log(result) // 'Goku'
You'd do:
function setHero(myHero) { myHero ??= 'Goku'; return myHero; } const result = setHero(); console.log(result) // 'Goku'
The motivation behind this is to reduce the extra step of needing to check values and then assign. Now all of that comes at once.
For other Falsy scenarios, you can use the good old || operator:
function setSalary(mySalary) { const minimum_salary = 1000; mySalary ||= minimum_salary; return mySalary; } const result = setSalary(); console.log(result) // 1000
This also applies to more complex scenarios:
const cybertruck = { topSpeedInKmh: 180, maximumBatteryLife: null, towingCapacity: undefined }; const defaultTopSpeed = 100; const defaultBatteryCapacity = '122.4 kWh' const defaultTowingCapacity = '11 000 pounds' cybertruck.topSpeedInKmh ||= defaultMaxSpeed; // takes first truthy value cybertruck.maximumBatteryLife ??= defaultBatteryCapacity; // avoids null cybertruck.towingCapacity &&= defaultTowingCapacity; // takes falsy value console.log(cybertruck.topSpeedInKmh); // 180 console.log(cybertruck.maximumBatteryLife); // '122.4 kWh' console.log(cybertruck.towingCapacity); // undefined
If a value evaluates to a truthy or a falsy value, you do not need to explicitly say that the outcome is either true or false.
if (value === true) { ... }
if (value) { ... }
const isGreaterThan = 10 > 1 ? true : false;
const isGreaterThan = 10 > 1;
const freeItem = clothes.find(item => item.price === 0);
const freeItem = clothes.find(item => !item.price); // { type: 'Shirt', color: 'Black', size: 'XL', price: 0 }
You can also explicitly convert a value into a boolean using a pair of exclamation marks.
const greeting = 'Hello'; const truthy = !!greeting; // True
const empty = ''; const falsy = !!empty; // False
Alternatively, you can use the Boolean() constructor:
const greeting = 'Hello'; const empty = ''; console.log(Boolean(greeting)); // True console.log(Boolean(empty)); // False
Author: