Author:
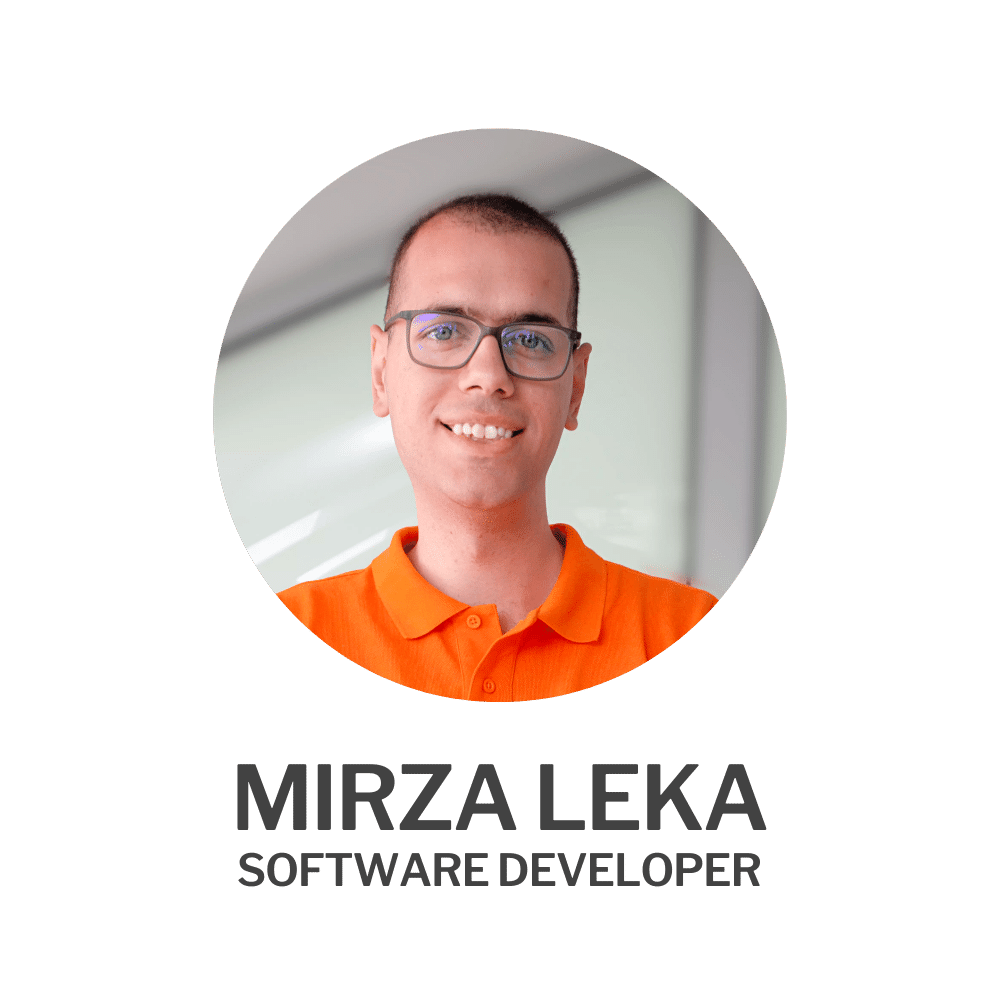
If you’ve ever used Node.js you might be aware that it comes with a powerful and easy-to-use REPL. An interactive shell for Node.js that lives in your terminal and can execute JS, import dependencies, and can even save sessions.
Node.js REPL (Read-Evaluate-Print Loop) is an interactive shell (similar to the console in the Browser) for the Node.js environment that allows us to write any valid Javascript code in it. This is used to test, evaluate, experiment, or debug code much easier and more accessible way.
In other words, it can read input from the user, evaluate, print the result, and accept another input. Repeat. Hence, Read-Evaluate-Print Loop.
Make sure you have Node.js installed on your machine. You can verify that by running node --version in your terminal. All of the commands you’ll see in this tutorial work both on Windows and Unix OS.
Let’s start simple. Open up your terminal (cmd) window and type node.
Here you can execute your favorite JavaScript commands. Simply type your command and hit Enter to evaluate.
Compiling JavaScript within REPL
from Pexels
Of course, you don’t need to write everything inline. You can create multiline statements with a combination of keys Shift + Enter.
Then finally hit Enter to evaluate.
Alternatively, you can type .editor which opens an editor mode, the likes of Vim. You create a new line with the Enter key. Once you finish writing, you can evaluate your code by pressing CTRL/CMD+D.
To clear a console, you can use the standard console cleaning command, (console.clear())
Finally, you can exit the REPL using CTRL/CMD+C
. . .
Another cool thing about REPL is that it gives you a list of commands available to you. Type the command and press period. After that hit the Tab key twice. This will print all properties and methods on that object.
console. tab tabCommands on console object within REPL
Commands on the instance of the Date object
This can be a useful reminder, since you probably don’t know all possible methods on strings, arrays, objects, etc. REPL also has IntelliSense, so as you type it will suggest an option to auto-complete.
This also works on any variables you create yourself.
Using the double Tab trick to preview custom-made fields.
You can type .help at any point in time to see what else is on offer.
. . .
Being Node.js REPL means you can also import any of the core modules, be it HTTP, File System, OS, or pretty much any other.
Likewise, you can make use of the double Tab trick to reveal all the methods available on the Node.js modules.
Also, you can just type one letter or part of the word and hit the Tab twice and Node.js will autocomplete all modules/objects/methods starting with that letter, e.g. type h and hit the Tab key twice.
. . .
You might be wondering if is it possible to use any of the external modules within the REPL, like Express, Day.js, Rx.js, or other?
Yes, of course! As long as the package is either server-side or cross-platform and is installed globally.
. . .
First of all, you can search through npm packages directly through your terminal (outside REPL). Type npm search <keyword> , where the keyword is the name or the description of the package you’re looking for.
Let’s search for Express, npm search express .
List of modules that are relevant to “Express”
Here we can see all the results matching with “Express”. You can also open the official page or GitHub repo of your desired package.
Another example of a search using a word relevant to the package. For example, there are a bunch of libraries on NPM that work with date formats. If you enter npm search date it will return libraries such as Moment, Date-Fns, Day.js, etc.
List of modules that are relevant with dates.
. . .
Now that we found the desired package we need to install it globally in order to use it within REPL.
You can then run npm list -g --depth=0 command to verify all of the packages that were installed globally.
Now go to the Node.js installation directory on your machine. You can find one by entering the command npm root -g or npm root --location=globaland this will return the correct path:
Then go to the into directory manually or via the command line (cd /usr/local/lib/node_modules ) and start a new REPL session inside this directory (node + Enter)
Importing third-party Node.js modules (NPM) into
REPL
. . .
The last piece of information I want to share today is that with REPL you save your progress and load it anytime.
To save, simply type .save <file-name.js> when finished. This will save the current REPL session in a file in the directory you were in.
Save the REPL session in a local file.
To resume, type .load <file-name.js>
Resume the REPL session from the previously saved file
. . .
Author: